Novelty API
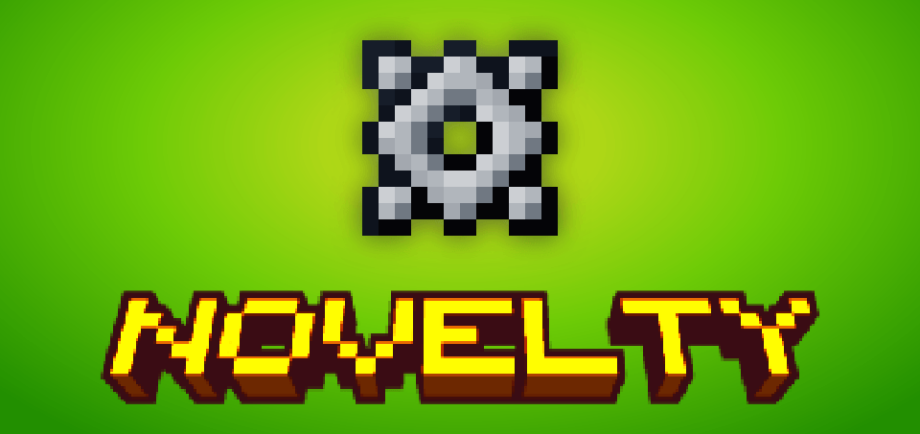
Novelty is a small addon that adds an additional equipment slot to the player. It adds 24 new slots, including a hat, face, necklace, 2 bracelets, 2 hands, 2 feet, a belt, a back, a spellbook, 2 charms, and 10 trinkets. This addon doesn't add any accessories; it is intended to be used by other addons.
Compatibility:
If you want to add your items to be compatible with the accessories slot, you have two options to make your own compatibility by using tags and commands or scriptings with an additional library.
Option 1: using custom tags
You can add those tags to your custom items:
"novelty:is_hat": Add item can be eqipped at hat slot,
"novelty:is_belt": Add item can be eqipped at belt slot,
"novelty:is_face": Add item can be eqipped at face slot,
"novelty:is_hand": Add item can be eqipped at hand slot,
"novelty:is_bracelet": Add item can be eqipped at bracelet slot,
"novelty:is_trinket": Add item can be eqipped at trinket slot,
"novelty:is_back": Add item can be eqipped at back slot,
"novelty:is_neckless": Add item can be eqipped at neckless slot,
"novelty:is_foot": Add item can be eqipped at foot slot,
"novelty:is_spellbook": Add item can be eqipped at spellbook slot,
"novelty:is_charm": Add item can be eqipped at charm slot
Player has equipped accessories slot will have tag "novelty:<item identifier>" example code:
Items/test_item.json:
{
"format_version": "1.20.80",
"minecraft:item": {
"description": {
"identifier": "example:item_hat",
"menu_category": {
"category": "equipment"
}
},
"components": {
"minecraft:icon": "brush",
"minecraft:tags": {
"tags": [
"novelty:is_hat"
]
},
"minecraft:max_stack_size": 1
}
}
}
And the command sample uses a tag selector:
execute at @a[tag=novelty:example:item_hat] run effect @s levitation
Option 2: using custom library scripting
Using a scripting library can allow you to define vanilla items to the accessories slot. By using scripting, you can also interact with the item, like seeing dynamic properties, durability, and also replacing the item. You can copy the library inside the script behavior pack at path "scripts/lib/NoveltyManager.js". Here is some little documentation:
Interface AccessoriesSlot:
Hat: "Hat"
Belt: "Belt"
Face: "Face"
Hand: "Hand"
Bracelet: "Bracelet"
Trinket: "Trinket"
Back: "Back"
Neckless: "Neckless"
Foot: "Foot"
Spellbook: "Spellbook"
Charm: "Charm"
Class NoveltyManager:
static registerAccessoriesItem(identifier, slot): return void
identifier: string, eg: "minecraft:apple"
slot: Interface AccessoriesSlot, eg: AccessoriesSlot.Hat
static registerAccessoriesItemFromTag(tag, slot): return void
tag: string, eg: "minecraft:is_sword"
slot: Interface AccessoriesSlot, eg: AccessoriesSlot.Hat
static getAccessories(player, slot, index = -1): return ItemStack | [ItemStack] | undefined
player: Class Player
slot: Interface AccessoriesSlot, eg: AccessoriesSlot.Hat
index: int, eg: 1
(index is suppose to select the index of the item, like hands with index 0 is left and 1 is right, also with trinket slot can select from 0 - 9, based on total amount of the slot. index -1 is mean to all of the items using array)
static getAccessoriesSlot(player, slot, index = -1): return ContainerSlot | [ContainerSlot] | undefined
player: Class Player
slot: Interface AccessoriesSlot, eg: AccessoriesSlot.Hat
index: int, eg: 1
(index is suppose to select the index of the item, like hands with index 0 is left and 1 is right, also with trinket slot can select from 0 - 9, based on total amount of the slot. index -1 is mean to all of the items using array)
static getAllAccessories(player): return [ItemStack]
player: Class Player
static getAllAccessoriesSlot(player): return [ContainerSlot]
player: Class Player
static setItem(player, slot, itemStack): return void
player: Class Player
slot: Interface AccessoriesSlot, eg: AccessoriesSlot.Hat
itemStack: Class ItemStack
Example code:
import { NoveltyManager, AccessoriesSlot } from "./lib/NoveltyManager";
import { system, world } from "@minecraft/server";
NoveltyManager.registerAccessoriesItem("minecraft:apple", AccessoriesSlot.Face);
system.runInterval(()=>{
for(let playerData of world.getPlayers()){
let face = NoveltyManager.getAccessoriesSlot(playerData, AccessoriesSlot.Face);
if(face != undefined && face.typeId == "minecraft:apple"){
playerData.runCommand("say using apple")
}
}
}, 5);
If you want to make it simple I recommend using option 1 but if you using more complex I recommend using option 2.
Images:
Updated on April 12
- Added accessories hover text when hovering to empty accessories slot
- Now accessories menu item will be back when player doesn't have an accessories menu
- Fixed when the item menu won't be back when inventory cleared
- Renamed "Neckless" to "Necklace" tag now using "novelty:is_necklace" that before is "novelty:is_neckless" but "novelty:is_neckless" still can be used for defining as a necklace